Diffie Hellman Exchange in C++ (Skills: Programming with C++, Mathematics (Cryptography))
The Purpose: To create a program that demonstrates the cryptographic functions behind the Diffie-Hellman Key exchange
Program would need to:
- Establish 2 users, Alice and Bob
- Have Alice and Bob introduce 2 values, p (a prime number) and q, a number which is a primitive root of p. The user will be prompted to input these values and the program will determine if p is a valid prime number.
- Alice and Bob choose individual private keys, kA and kB. User will input these values.
- Alice computes public key, pubkA: qkA mod p
- Bob computes public key, pubkB: qkB mod p
- A shared value, s, will be achieved by calculating sA (for Alice) and sB (for Bob)
- sA = pubkBkA mod p
- sB = pubkAkB mod p
- Program will output kA and kB and will check to see if kA and kB are equal. If they are, the user will be informed that the Diffie Hellman exchange has been completed.
- Comments throughout will show the purpose and logic of each section.
Code is as follows:
#include <cmath>
#include <iostream>
using namespace std;
// function for finding the values with modular math.
// due to inherent limits within C, long long must be used as the int type
// in order to ensure that exponentiation doesn't overflow with values too high.
// Despite this, there is still a limit within this program, so small numbers
// should be used for private keys kA and kB, pubkA and pubkB.
long long int modmath(long long int x, long long int y, long long int p1)
{
if (y == 1)
return x;
else
return (((long long int)pow(x, y)) % p1);
}
// Function for checking the status of numbers entered to see if they're prime.
// The variable "p" needs to be prime for Diffie Hellman exchange.
// The function works well and is an easy addition to the program to ensure
// that no improper inputs are given
bool PrimeCheck(int a)
{
if (a<= 1)
return false;
for (int i =2; i <= a / 2; i++)
if (a % i == 0)
return false;
return true;
}
// Main function
int main()
{
long long int p, q, x, y, p1;
long long int kA, kB, pubkA, pubkB;
long long int sA; int sB;
cout << "Please enter shared values, p and q, required for the Diffie Hellman Exchange" << "\n";
cout << "Separate by space. 'p' should be a prime number, q should be a primitive root of p" << endl;
cin >> p >> q;
// check for prime numbers using primecheck function
if (PrimeCheck(p) == false){
cout << "p needs to be a PRIME number. \nPlease enter a prime number p" << endl;
cin >> p;
}
cout << "You've selected the values " << p << " for p, and " << q << " for q." << endl;
// ask Alice and Bob for their private keys, pkA and pkB, which are to be whole numbers.
cout << "Choose a private key for Alice" << "\n" << "This number needs to be a whole number" << endl;
cin >> kA;
cout << "Choose a private key for Bob (this needs to be a whole number)" << "\n";
cin >> kB;
cout << "Awesome. Alice's private key is " << kA << " and Bob's private key is " << kB << endl;
// calculate pubkA and pubkB using modmath function and values inputted from the user
pubkA = modmath(q, kA, p);
pubkB = modmath(q, kB, p);
cout << "Alice's public key : " << pubkA << "\n" << "Bob's public key: " << pubkB << endl;
// calculate sA and sB, the values that Alice and Bob ultimately get.
// These 2 values should be the same
sA = modmath(pubkB, kA, p); // something wrong with the math here
sB = modmath(pubkA, kB, p);
// debugging to check math :::: cout << sA << "\n" << sB << endl;
//
if (sA == sB){
cout << "shared value sA for Alice is " << sA << " and the value sB for Bob is " << sB << "\nsA is equal to sB,";
cout << "the Diffie Hellman Exchange was successful" << endl;
} else{
cout << "Something went wrong. p or q were either too large\n or " << q << " is likely not a primitive root of " << p << endl;
}
return 0;
}
When compiled, program gave the following output:
Please enter shared values, p and q, required for the Diffie Hellman Exchange
Separate by space. 'p' should be a prime number, q should be a primitive root of p
17 5
You've selected the values 17 for p, and 5 for q.
Choose a private key for Alice
This number needs to be a whole number
3
Choose a private key for Bob (this needs to be a whole number)
7
Awesome. Alice's private key is 3 and Bob's private key is 7
Alice's public key : 6
Bob's public key: 10
shared value sA for Alice is 14 and the value sB for Bob is 14
sA is equal to sB,the Diffie Hellman Exchange was successful
As can be seen, the program was successfully able to complete the cryptographic functions behind the Diffie-Hellman Key Exchange given user variables.
HTTP Finder: Developing A Cybersecurity Pen Testing Tool (Skills: Programming with Python, Problem solving, network security, Linux system security)
HTTP is an outdated and insecure transfer protocol used by websites. In the current year, 2024, it is highly recommended to use HTTPS as the transfer protocol. There are still many websites that use HTTP, which can cause security issues for both the website in question and the users accessing them.
To find sites that use HTTP (For research and penetration testing purposes), I used data aggregated from a crawler called “crawler.ninja”. A crawler is an automated program that goes through the internet and indexes the content it finds. As this program crawls across the web, it is often (cleverly) called a spider. Crawlers can collect links, code, and other website content such as security information. The ability of this crawler makes it perfect for this project.
METHOD:
The first step of this task was to access the data aggregated by this crawler. Visible (in purple) is the data that we need.
After opening the file “http-sites.txt”, a massive file with thousands upon thousands of http sites is shown
The file was then saved to my local linux system. It is clear, despite the dates shown on crawler.ninja, that this information is out of date, and that the date next to the files is simply the “date accessed” information. More will need to be done to reliably tell that these sites are valid HTTP sites.
I needed to create a simple program that would sort through these websites to check if they were indeed valid HTTP sites, as some of these sites, when tested in google, were completely offline. Normally, when an HTTP site is accessed in google, the user gets a “Not Secured” flag in the search bar.
The program would need to:
- Format the raw data downloaded via text file into data that a program can read
- Apply the proper website formatting to the site (adding ‘http://’)
- Automatically test each website and print out results that are online and return with an HTTP request.
I chose to use Python for this program out of simplicity. The first program was one that would format the raw data from the file named “crawlerninja.txt”, remove the index numbers in the beginning of each line, and output “websites.txt”
Python was chosen as my language of choice for this project due to the need of the program to scrape information from files, format them, and then check their status to see if said sites are online. Code is as follows:
#the crawler file downloaded from the website can't really be used in this format
#this file will remove the numbers and print a website on each line
def extract_every_other_word(input_text):
words = input_text.split()
every_other_word = '\n'.join(words[i] for i in range(0, len(words), 2))
return every_other_word
def main():
# input file
input_file_path = 'crawlerninja.txt'
# output file
output_file_path = 'websites.txt'
try:
with open(input_file_path, 'r') as input_file:
input_text = input_file.read()
every_other_word_text = extract_every_other_word(input_text)
with open(output_file_path, 'w') as output_file:
output_file.write(every_other_word_text)
print(f"Every other word extracted and saved to {output_file_path}")
except FileNotFoundError:
print(f"Error: File '{input_file_path}' not found.")
except Exception as e:
print(f"An error occurred: {e}")
if __name__ == "__main__":
main()
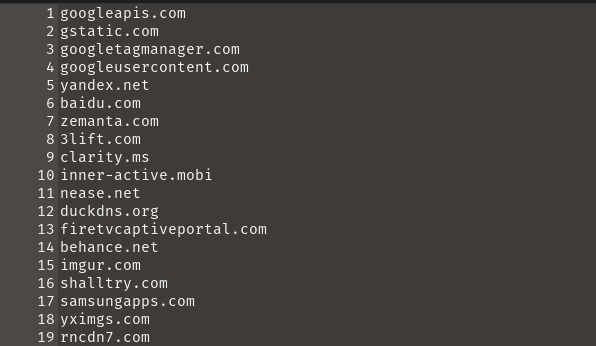
The second program would take this fixed data and run through and test each site, ensuring that each site is still online and connects via HTTP by sending status codes. The initial program that I used printed both “online” and “offline” next to each website depending on the status code that was received. To clean it up I decided to only print the online sites. Due to the size of the file, testing the status of these sites would take hours, so I decided to limit it to only the first 300 processes. Output continues below the program with 159 results out of 300 tested.
import requests
def check_website_status(url):
try:
response = requests.get(url, timeout=5)
if response.status_code == 200:
return True
except (requests.ConnectionError, requests.Timeout):
pass
return False
def main():
# input file
input_file_path = 'websites.txt'
# output file
output_file_path = 'HTTPwebsites2024_online.txt'
# Only process first 300 websites (otherwise this would take all day)
max_websites = 300
#output one website per line
try:
with open(input_file_path, 'r') as file:
websites = file.read().splitlines()[:max_websites]
with open(output_file_path, 'w') as output_file:
for website in websites:
# add 'http://' to the front of the website names
full_url = f"http://{website}"
# only write websites to file that are actually online
if check_website_status(full_url):
output_file.write(f"{full_url}: online\n")
print(f"Success: Written to '{output_file_path}'")
except FileNotFoundError:
print(f"Error: File '{input_file_path}' not found.")
except Exception as e:
print(f"An error occurred: {e}")
if __name__ == "__main__":
main()
With the information from this program, we can see 159 websites that are currently still up and using HTTP. In the initial crawler.ninja data, there were several adult sites which I will be omitting from the list.
Insecure HTTP sites (Click to expand)
- http://yandex.net: online
- http://baidu.com: online
- http://3lift.com: online
- http://nease.net: online
- http://duckdns.org: online
- http://firetvcaptiveportal.com: online
- http://behance.net: online
- http://imgur.com: online
- http://samsungapps.com: online
- http://rncdn7.com: online
- http://google.cn: online
- http://adrta.com: online
- http://pendo.io: online
- http://genius.com: online
- http://cedexis-test.com: online
- http://rayjump.com: online
- http://arxiv.org: online
- http://grserver.gr: online
- http://adgrx.com: online
- http://eepurl.com: online
- http://abovedomains.com: online
- http://quickconnect.to: online
- http://superhosting.bg: online
- http://uchi.ru: online
- http://bttrack.com: online
- http://mmechocaptiveportal.com: online
- http://ddns.net: online
- http://166.com: online
- http://acsechocaptiveportal.com: online
- http://nakheelteam.cc: online
- http://alipaydns.com: online
- http://avsxappcaptiveportal.com: online
- http://lwsdns.com: online
- http://ripn.net: online
- http://hollywoodbets.net: online
- http://cmoa.jp: online
- http://lausd.net: online
- http://lxtgame.com: online
- http://ucweb.com: online
- http://onlar.az: online
- http://jwpltx.com: online
- http://ad-delivery.net: online
- http://dnsdomen.com: online
- http://liveperson.net: online
- http://sberbank.ru: online
- http://gda.pl: online
- http://cedexis.com: online
- http://inleed.net: online
- http://adsco.re: online
- http://heytapmobi.com: online
- http://undertone.com: online
- http://pndsn.com: online
- http://cbr.ru: online
- http://redditmedia.com: online
- http://skysrt.com: online
- http://armgs.team: online
- http://izatcloud.net: online
- http://dfaklj.tech: online
- http://micpn.com: online
- http://lifehacker.com: online
- http://streamtheworld.com: online
- http://amagi.tv: online
- http://padlet.com: online
- http://otm-r.com: online
- http://tabletcaptiveportal.com: online
- http://livedns.co.uk: online
- http://webflow.com: online
- http://imolive2.com: online
- http://clean.gg: online
- http://faqs.org: online
- http://attdealer.biz: online
- http://sureserver.com: online
- http://ibosscloud.com: online
- http://imoim.net: online
- http://storygize.net: online
- http://cygwin.com: online
- http://hath.network: online
- http://dvr163.com: online
- http://ekatox.com: online
- http://gifshow.com: online
- http://com.com: online
- http://ctnsnet.com: online
- http://histats.com: online
- http://zuimeitianqi.com: online
- http://streamtheworld.net: online
- http://sina.com: online
- http://qvdt3feo.com: online
- http://crcldu.com: online
- http://evolutionstarbucks.com: online
- http://parkingcrew.net: online
- http://paytm.com: online
- http://vapp-servers.com: online
- http://biglobe.ne.jp: online
- http://retargetly.com: online
- http://dailyinnovation.biz: online
- http://meituan.net: online
- http://kikakuya.ne.jp: online
- http://zlib.net: online
- http://av380.net: online
- http://bizport.cn: online
- http://tru.am: online
- http://fireoscaptiveportal.com: online
- http://insurads.com: online
- http://cdn4image.com: online
- http://pcre.org: online
- http://plground.live: online
- http://gzip.org: online
- http://heytapdownload.com: online
- http://mihanwebhost.com: online
- http://feednews.com: online
Addressing Cybersecurity Concerns in Smart Cities: A research project (Skills: 2D Modeling, Research, Writing Skills, Cybersecurity Risk Management, Vulnerability Research)
Participated in a group research project where the challenges of securing a “Smart City”, specifically the transport and transit sectors, were proposed. I was tasked with researching topologies of basic smart cities and IoT devices that helped interconnect them, creating models of a smart city, and then implementing security measures into the city and determining where they might fail. Proposed attack vectors were explored, as well as mitigation techniques.
Below is a model of the smart city that I created for this project with Microsoft Visio
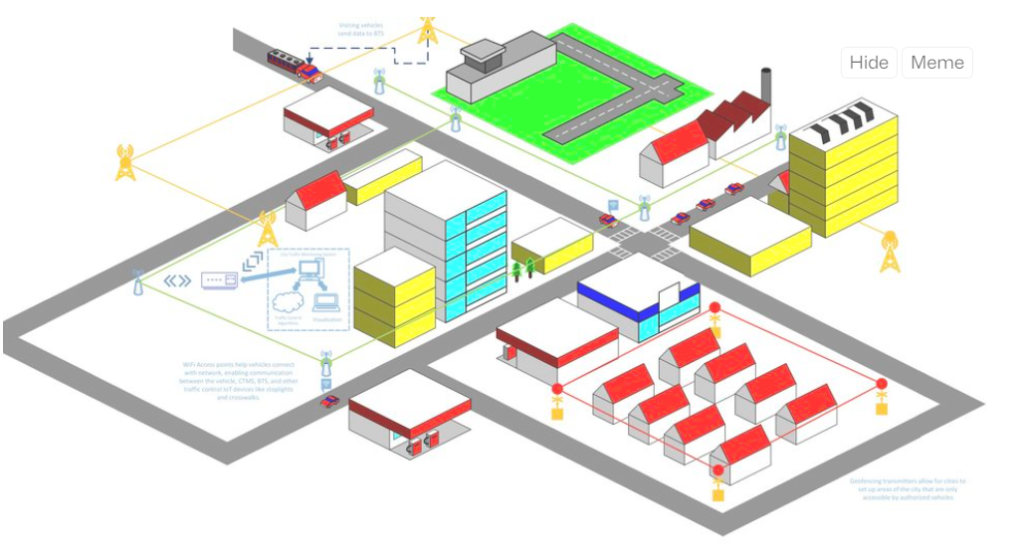
We also explored other avenues of attack that could effect the software withing IoT devices such as sensors, WiFi Hotspots, and geofences. By showing the working mechanisms of a “Supply Chain” attacks and “Man in the Middle” attacks through use of models and diagrams, we were able to clearly articulate how these attacks could impact a smart city.
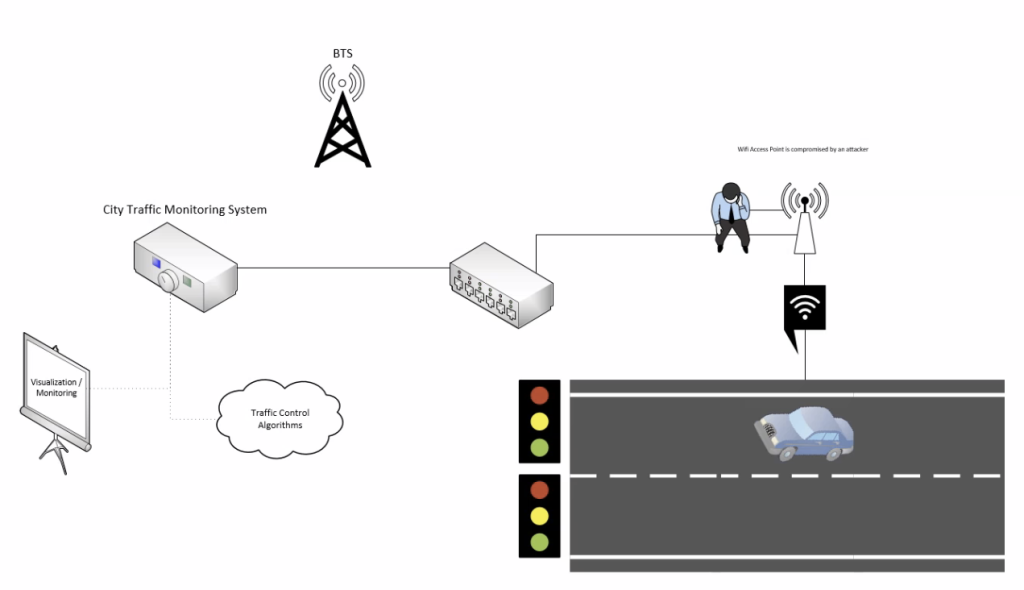
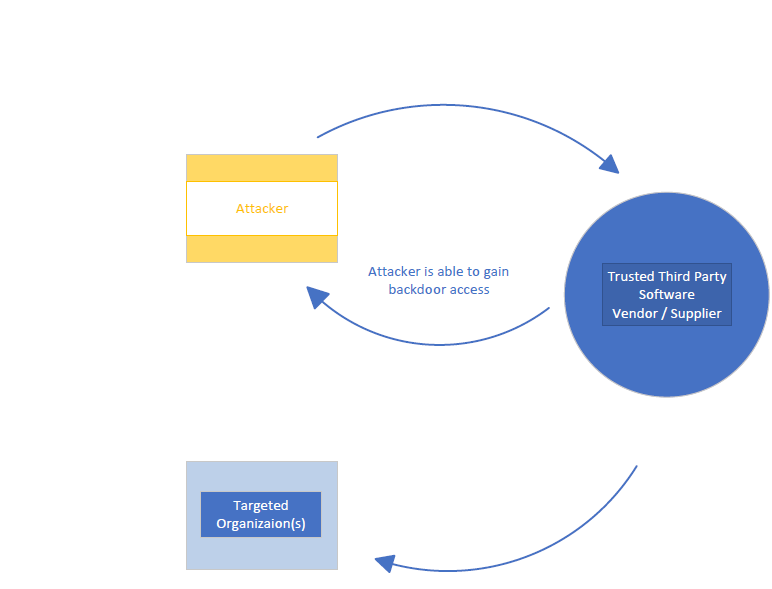
From our research, we came to the conclusion that the supply chain, unauthorized access, and Man in the Middle attacks were most likely to cause damage to a smart city. The best mitigation techniques were implementing “Secure by Design” principles. End to end encryption should be used to make it more difficult for Man in the Middle attacks to harvest data or spoof inputs.
A link to said paper can be found below
Malware Analysis: EternalBlue
EternalBlue was one of the most destructive exploits in the modern day, and the origins of it still remain unknown to many. I wrote a paper which showed the intricate inner workings of EternalBlue, devices that the exploit effected, and some known fixes for the exploit (Skills: Malware Analysis, Security Research, Networks)