Here’s some Java code I created! What started as school projects quickly turned into something much more, as I had a lot of fun working on them. On this page, I’ll showcase three of my favorite projects:
- Shrek-Themed ATM: For my first project, I created an ATM inspired by Shrek!
- Wizard Grade Book: My second project is a grade book made specifically for wizards.
- Flight Reservation Machine: Finally, I built a flight reservation system as my third project.
Shrek-Themed ATM
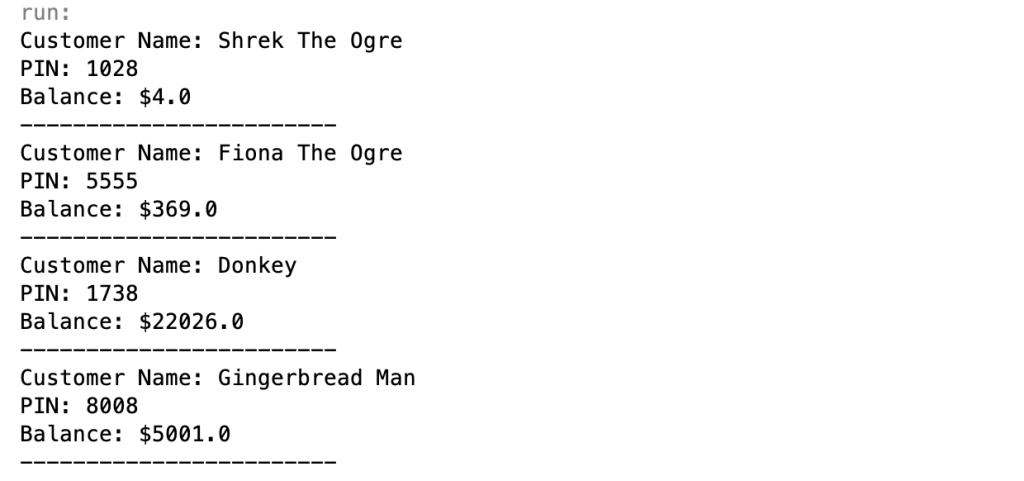
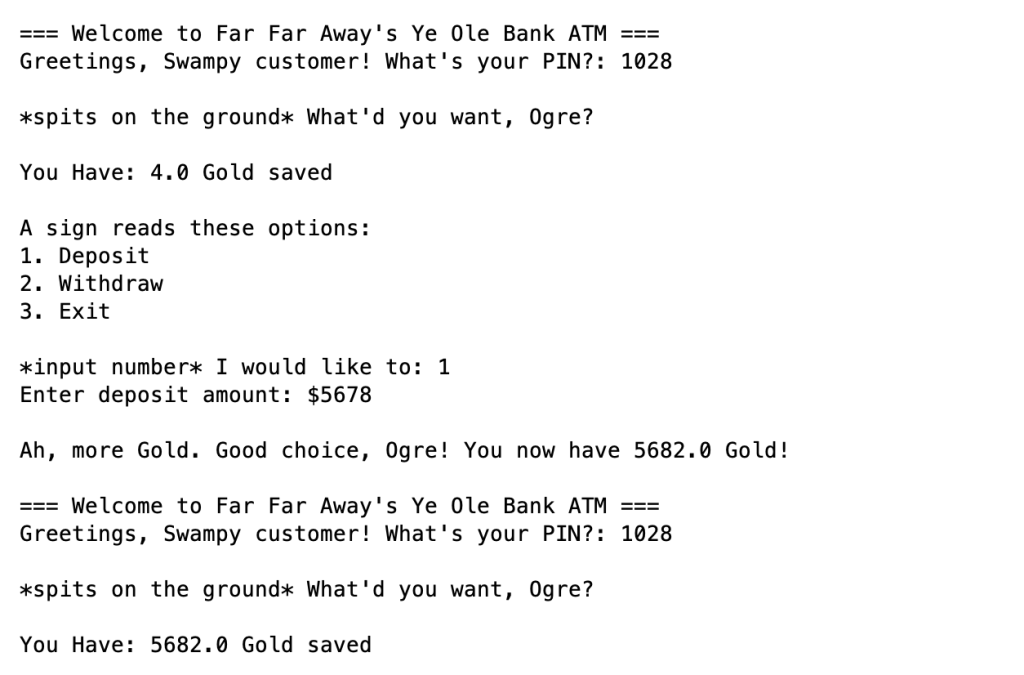
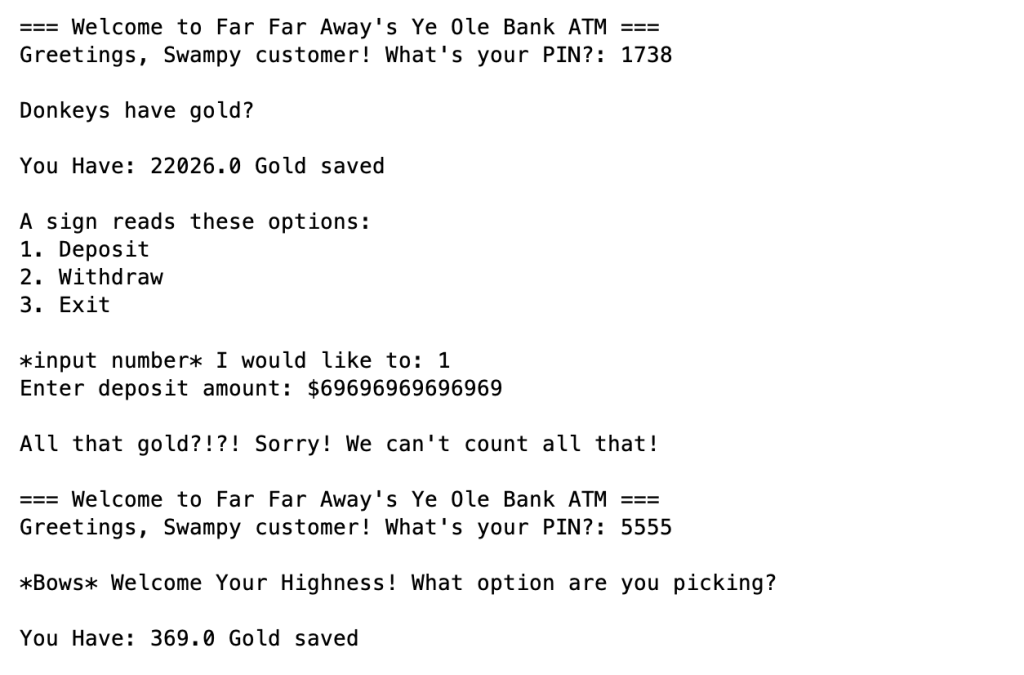
Shrek Code
// Method for withdrawals and teller phares
public void withdraw(double amount) {
if (amount > 0 && amount <= Gold) {
Gold -= amount;
if (getName().equals("Shrek The Ogre")) {
System.out.println("\nHere, take your measly " + amount + " Gold.");
} else if (getName().equals("Donkey")) {
System.out.println("\nThe teller whispers: I guess they do. \nHere you go!: " + amount + " Gold!");
} else if (getName().equals("Fiona The Ogre")) {
System.out.println("\nHere you are your Highness!: " + amount + " Gold! Would you like anything else?");
} else if (getName().equals("Gingerbread Man")) {
System.out.println("\nHere ya go Gingy!: " + amount + " Gold! anything else?");
} else {
System.out.println("\nWithdrawal successful. Your new balance is: " + Gold + " Gold!");
}
} else {
if (getName().equals("Shrek The Ogre") && Gold - amount >= 0) {
System.out.println("\nStop wasting my time, Ogre! You don't have that much Gold.");
} else if (getName().equals("Donkey")) {
System.out.println("\nGet outta here! You don't need that much Gold, Donkey!");
} else if (getName().equals("Fiona The Ogre")) {
System.out.println("\nI'm sorry princess but we can not provide you with that amount.");
} else if (getName().equals("Gingerbread Man")) {
System.out.println("\nHaHa! Nice Try. But let's be serious!");
} else {
System.out.println("\nInvalid withdrawal amount or insufficient coins.");
}
}
}
Wizard Grade Book
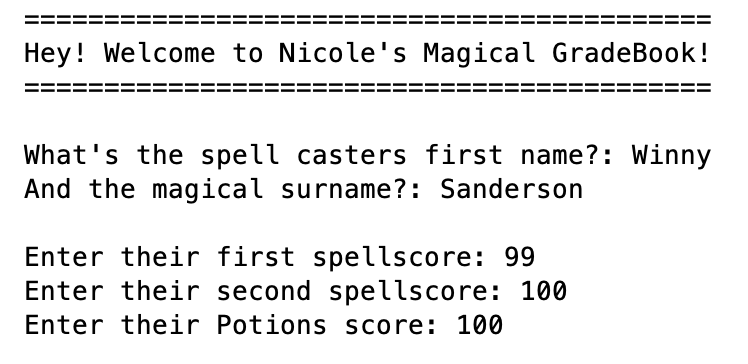
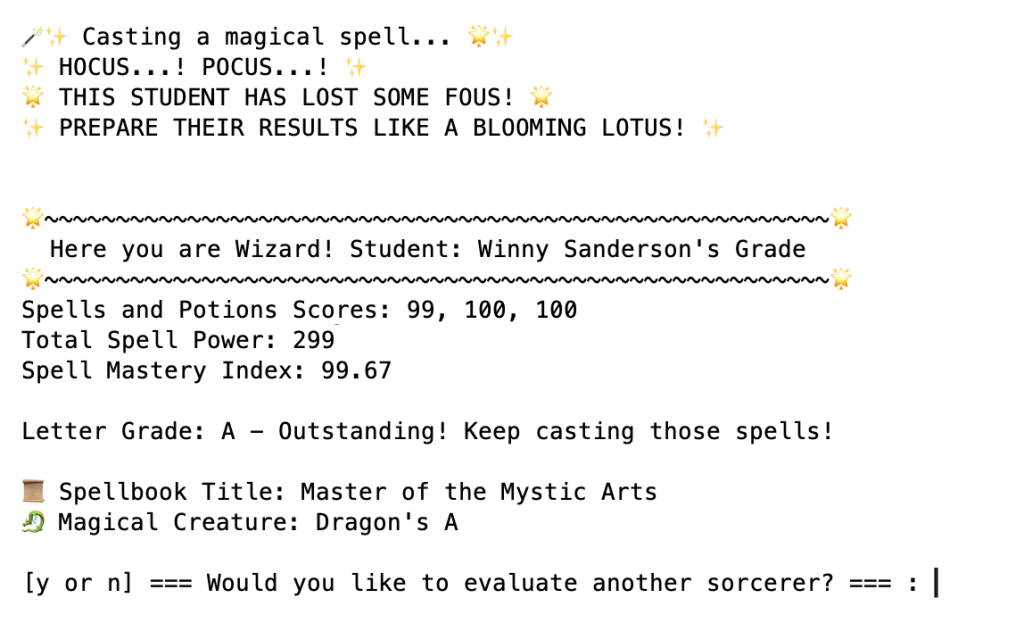
// Helper method to add up all the scores. Math is fun!
public static int calculateTotal(int score1, int score2, int score3) {
return score1 + score2 + score3;
}
// Helper method to find the average. More math magic!
public static double calculateAverage(int total) {
return (double) total / 3;
}
// Helper method to get the super cool letter grade!
public static char determineLetterGrade(double average) {
// Let's play with letters and grades!
if (average >= 90) {
return 'A';
} else if (average >= 80) {
return 'B';
} else if (average >= 70) {
return 'C';
} else {
// If it's not an A, B, or C, then it's an F. Oops!
return 'F';
}
}
Flight Reservation Machine
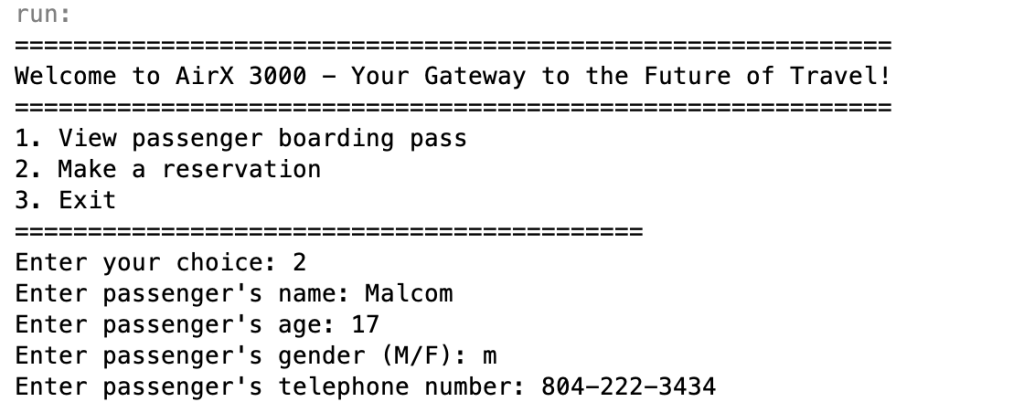
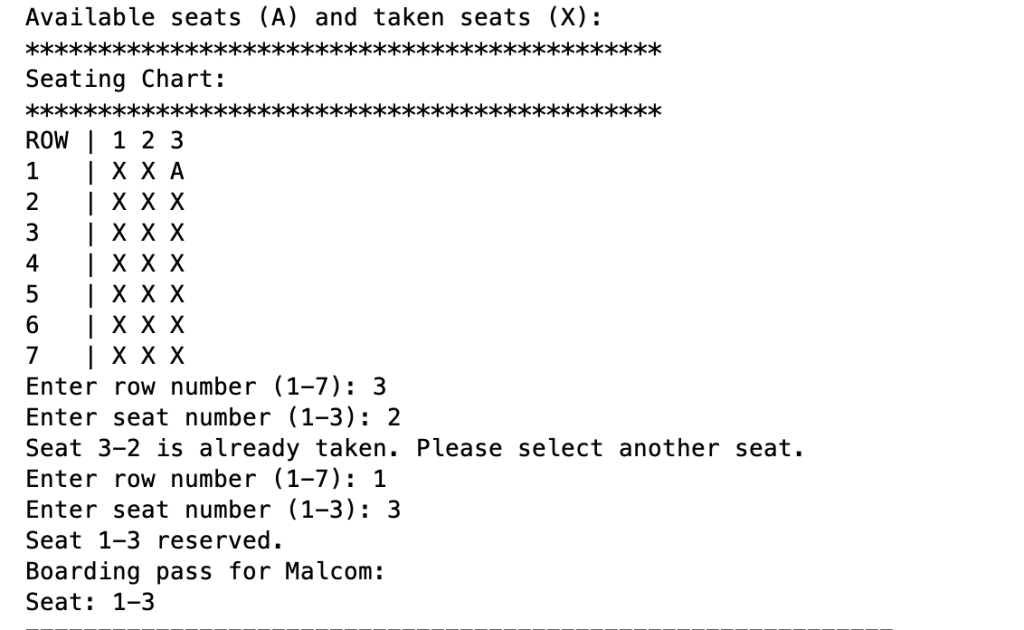
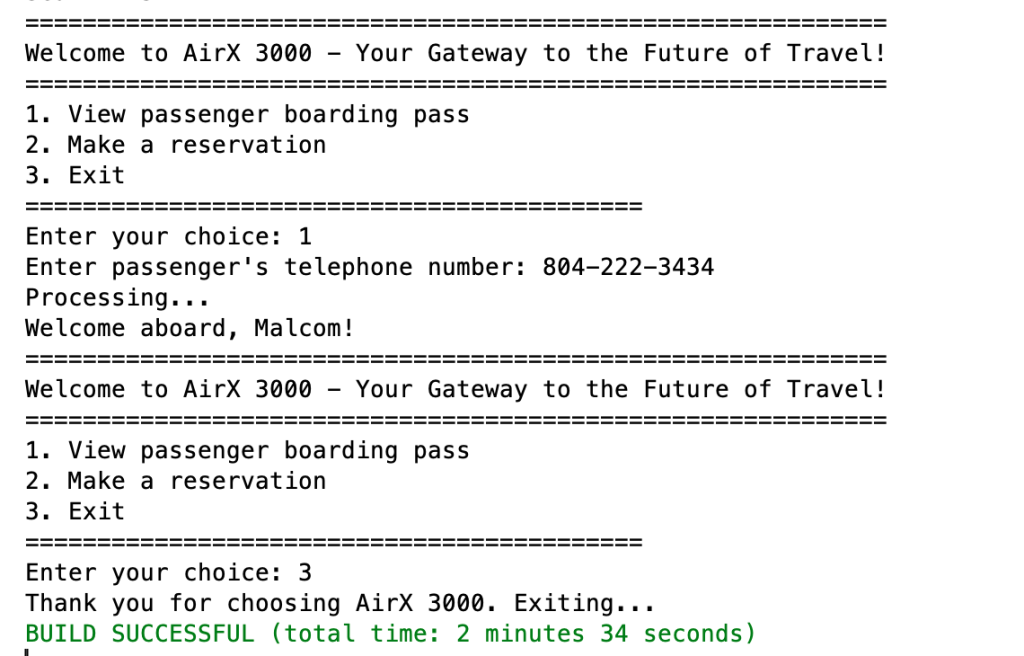
// Flight class to manage reservations and boarding passes
public class Flight {
// Static variables defining the futuristic aircraft
private static final int ROWS = 7; // Number of rows in the aircraft
private static final int SEATS_PER_ROW = 3; // Number of seats per row
private static char[][] seatingChart = new char[ROWS][SEATS_PER_ROW]; // Seating arrangement matrix
private static int seatsTaken = 0; // Number of seats already reserved
private static ArrayList<Passenger> passengerList = new ArrayList<>(); // List of passengers
// Initializing the seating chart of the aircraft
static {
// Creating a state-of-the-art seating arrangement
// In the AirX 3000, passengers are seated in a luxurious 7x3 configuration
// with unparalleled comfort and style.
// The future is here!
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < SEATS_PER_ROW; j++) {
if (i == 0 && j == 2) {
seatingChart[i][j] = 'A'; // 'A' for available seat
} else {
seatingChart[i][j] = 'X'; // 'X' for unavailable seat
}
}
}
}