Basic Cybersecurity Programming & Networking
This course introduces students to the logic used to develop solutions to common problems in the
computer science field using a contemporary high-level programming language, such as Python. These step-by-step detail solutions are called algorithms and serve as the basic solution in the area of Cybersecurity. Problem-solving, critical thinking, and programming techniques are emphasized
throughout the course. Topics include Introduction to Cybersecurity, Computer Network, program
logic, and file management.
Objective 1: Understanding the basic concepts of Networking and its importance
Objective 1.1: Learn about the importance of networking in Cybersecurity
Objective 1.2: Learn about network components and topologies
Objective 1.3: Learn to design and draw network using Microsoft Visio
Objective 2: Types of networks and Network Model
Objective 2.1: Learn about types of networks – LAN, MAN, WAN, PAN, Wi-Fi, etc. Objective
2.2: Network models – OSI, TCP/IP, etc.
Objective 3: TCP/IP model and its protocols
Objective 3.1: Compare and contrast the TCP/IP and the OSI reference model Objective
3.2: Learn about different layers protocols and their uses
Objective 3.3: Learn about IP (Internet Protocol) addresses and their classification
Objective 3.4: Learn about the importance of subnetting
Objective 4: Simulation using Cisco Packet Tracer and Networking Commands
Objective 4.1: Introduction to Cisco Packet Tracer
Objective 4.2: Learn about live simulation using Cisco Packet Tracer, repeaters, hubs, switches, bridge,
router, and gateway.
Objective 5: Network administration on Windows 8 Server
Objective 5.1: Learn about servers and adding user roles as an Administrator
Objective 5.2: Learn to use Virtual Machine and install Windows 8 server
Objective 6: Network Security and Malware
Objective 6.1: Learn the role of Malware (Malicious Software) in Network Security
Objective 6.2: Learn about the families of malware
Objective 7: Introduction to Computer Systems, algorithm, and coding (Programming)
Objective 7.1: Learn about computer Systems and their components
Objective 7.2: Learn about the availability of different general-purpose programming languages
Objective 7.3: Learn about an approach to developing algorithms and their flow chart Objective
7.4: Learn the importance of programming in Cybersecurity
Objective 8: Programming development environment, Python3, and IDE (Integrated
Development Environment)
Objective 8.1: Learn to install IDE and Python3
Objective 8.2: Learn to develop the first program in Python – syntax, data types, variables in Python
Objective 8.3: Learn about taking input, getting output, and typecasting.
Objective 9: Conditional Statements and logical operators
Objective 9.1: Learn about control flow in the script
Objective 9.2: Learn to apply if-else conditional statements for real-world problems.
Objective 9.2: Learn to apply logical and mathematical operators in if-else statements.
Objective 10: Loop statements
Objective 10.1: Learn to apply loop statements to real-world problems
Objective 10.2: Learn about finite (for loop) and infinite (while) loops
Objective 10.3: Learn to develop Python script using loop and conditional statements.
Objective 11: Python Data structures – Lists, Dictionary, and Tuple
Objective 11.1: Learn about Lists, methods, and applications
Objective 11.2: Learn about dictionaries, methods, and applications
Objective 11.3: Learn about tuples, methods, and applications
Objective 12: Developing functions for code reusability
Objective 12.1: Learn about functions to achieve repetitive tasks by creating functions in Python
Objective 12.2: Learn about function arguments
Objective 12.3: Learn about defining and calling a function
Objective 13: Introduction to file handling and Object-Oriented programming (OOP)
Objective 13.1: Learn to open, read, and write a text file
Objective 13.2: Develop an idea about object-oriented programming
Objective 14: Client-server architecture and socket programming
Objective 14.1: Learn about client-server architecture
Objective 14.2: Learn about socket programming
Objective 14.3: Learn to develop secure communication between client(s) and server in the network
using socket.
COURSE MATERIAL
Python (*.py) Language Editor
• Access to Text Editor: Python IDLE (Integrated Development and Learning Environment),
PyCharm, Visual Studio Code, Sublime Text, Atom, Emacs, etc.
• Online editor: https://replit.com
Assignments
String Assignment
Purpose: This assignment provides you with a deeper understanding and practical experience of
strings.
Requirements & Submission:
1. The answers of this assignment must be submitted electronically on canvas.
2. Some answers require more details than just what we had discussed in lecture slides.
Question (1) (Total: 100 points)
Write a program to count the number of vowels and consonants in a given string.
Ex.: string =“Python is fun”
Consonants:8
Vowels: 3
NOTE: Do not use Python string count method
Question (2) (Total: 100 points)
Write a program to count the occurrence of a given character in a string?
Ex.: string=“Coding is fun” character=’i’
Count=2
NOTE: Do not use Python string count method
Question (3) (Total: 100 points)
Write a program to remove a given character from String?
Ex.: string=“Coding is fun” character=’i’
output=“Codng s fun”
Note: Do not use Python string replace method
Question (4) (Total: 100 points)
Given a string, create a new string without vowels and print that string.
Ex.: string=“Coding is fun”
Output=”Cdng s fn”
Note: Do not use Python string replace method
Vowel Counter Python Program:
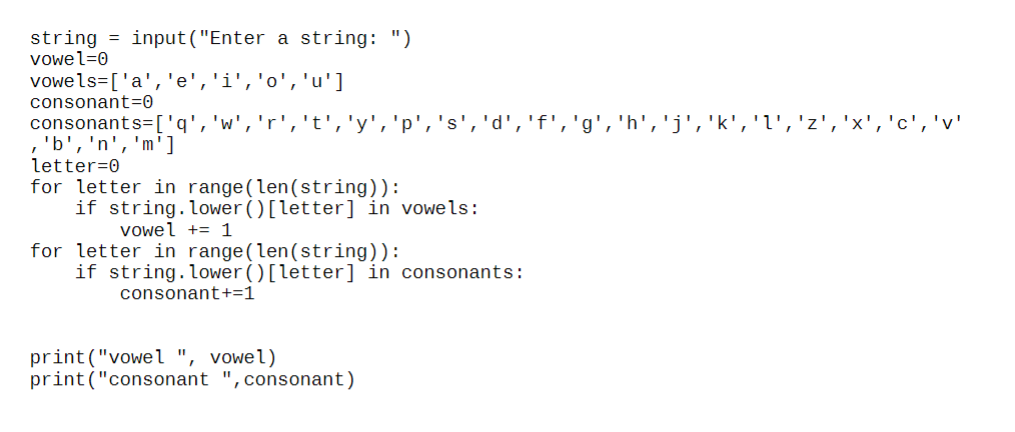
Character Counter Python Program:
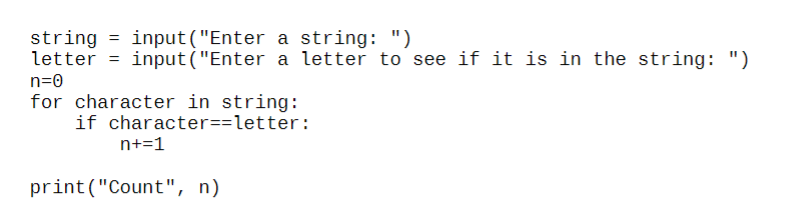
Removing a character from a string Python Program:
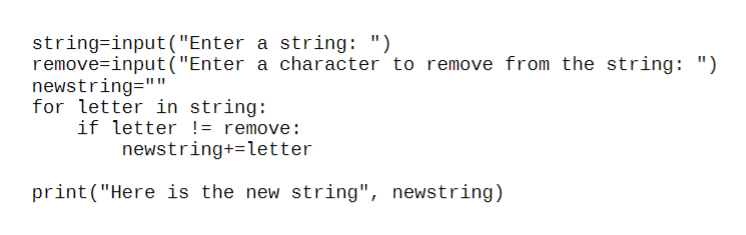
Removing Vowels Python Program:
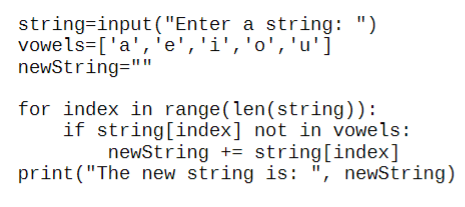
If Statement Assignment
Purpose: This assignment provides you with a deeper understanding and practical experience of
fundamental concepts of Python and conditional statements.
Requirements & Submission:
1. The answers of this assignment must be submitted electronically on canvas.
2. The Python code must be submitted for all questions except Question 3.
3. Some answers require more details than just what we had discussed in lecture slides.
Question (1) (Total: 100 points)
Write a python script to find the greatest of two numbers
• Take Two numbers as input
• Use if -else condition to check the greatest of the two numbers
• Print the greatest number.
You need to submit the *.py file.
Question (2)
(a) (100 points) Nancy teaches a science class, and her students are required to take three tests.
She wants to write a program that her students can use to calculate their average test score.
She also wants the program to congratulate the student enthusiastically if the average is
greater than 95.
Here is the algorithm in pseudocode:
Get the first test score
Get the second test score
Get the third test score Calculate the average
Display the average
If the average is greater than 95: Congratulate
the user
Write a python program for the above pseudocode.
(b) (100 points) Chris owns an auto repair business and has several employees. If any
employee works over 40 hours a week, he pays them 1.5 times their regular hourly pay
rates for all hours over 40. He has asked you to design a simple payroll program that
calculates an employee’s gross pay, including any overtime wages.
Greatest Number Python Program
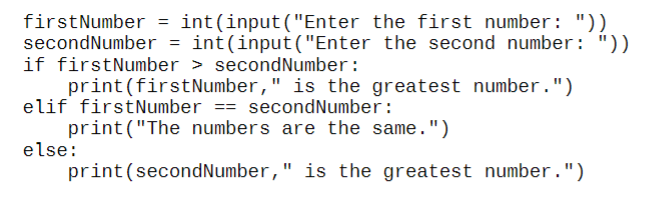
Gross Pay Python Program
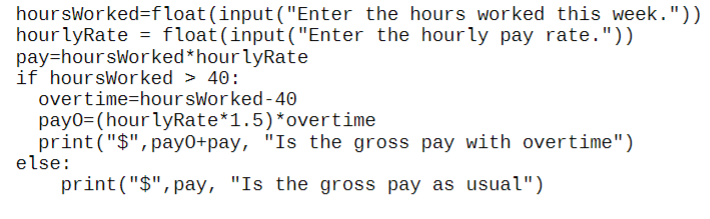
Test Scores Python Program
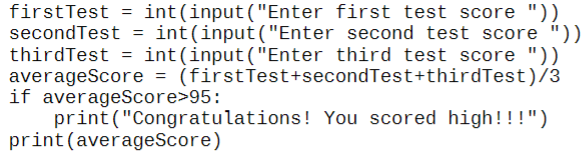
Loan Approval Python Program
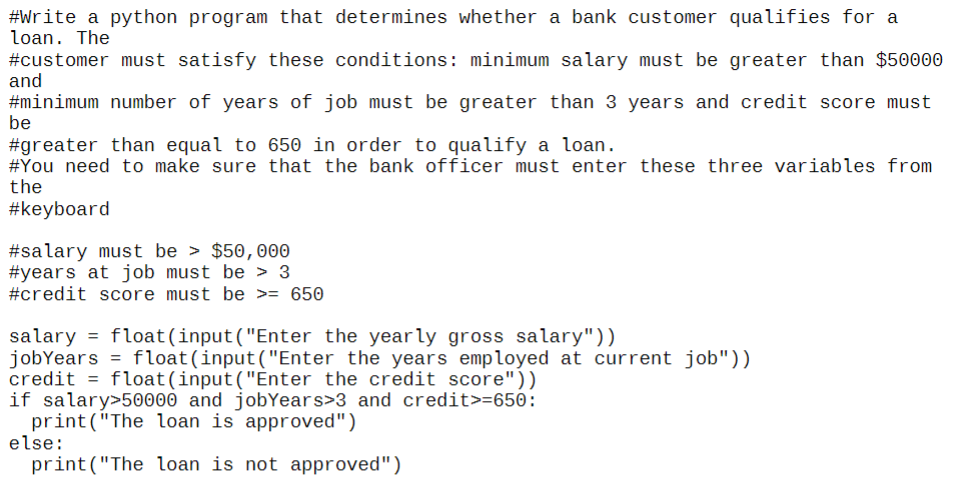
For Loop Assignment
Purpose: This assignment provides you with a deeper understanding and practical experience of
fundamental concepts of Python and conditional statements.
Requirements & Submission:
1. The answers of this assignment must be submitted electronically on canvas.
2. Some answers require more details than just what we had discussed in lecture slides.
Question (1) (Total: 100 points)
Write a program in python to read integer n and then find the sum of the series [x
– x^3 + x^5 + ……].
Question (2) (Total: 100 points)
Write a program in python to read 10 numbers from the keyboard and find their
sum and average.
Question (3) (Total: 100 points)
Write a program in python to read integer n and then display the multiplication table
for the given integer.
Question (4) (Total: 100 points)
Write a program in python to read n integer and then display the n terms of a
harmonic series and their sum.
1 + 1/2 + 1/3 + 1/4 + 1/5 … 1/n terms
Question 1 Python Program:
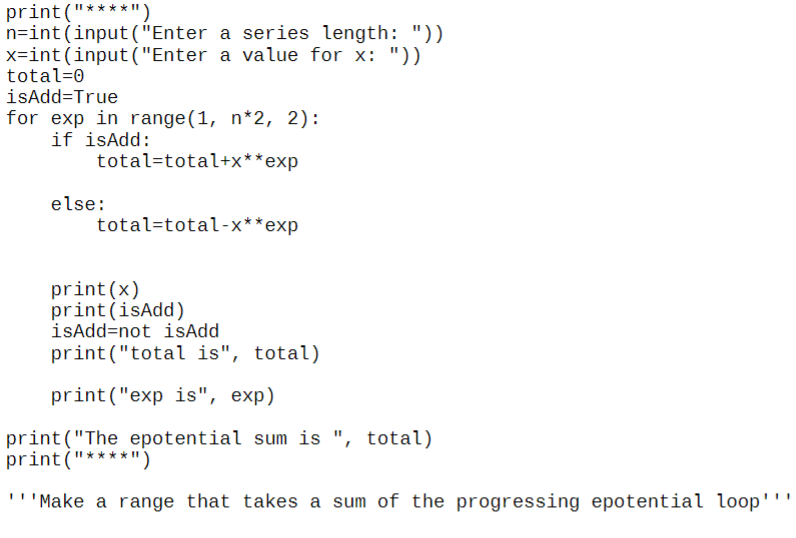
Question 2 Python Program:
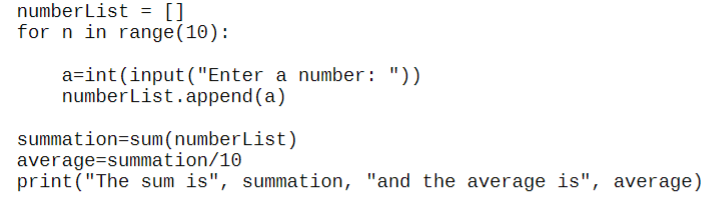
Question 3 Python Program:
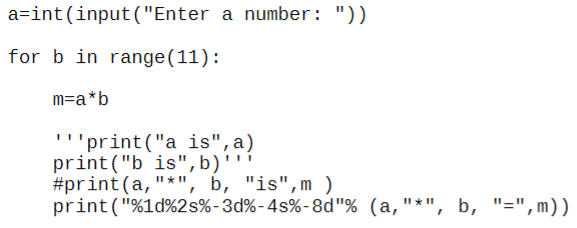
Question 4 Python Program:
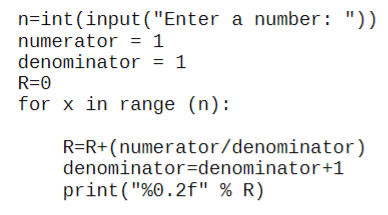
Files Assignment
Purpose: This assignment provides you with a deeper understanding and practical experience of Files.
Requirements & Submission:
1. The answers of this assignment must be submitted electronically on canvas.
2. Some answers require more details than just what we had discussed in lecture slides.
Question (1) (Total: 100 points)
Write a program to copy a file into another file duplicating each line.
Ex.:
input File:
Python is fun.
I like programming.
Output File:
Python is fun.
Python is fun.
I like programming.
I like programming.
Question (2) (Total: 100 points)
Write a program to read in a file containing numbers. Each line is composed of only one single number.
You should create an output file containing the square of each number from the first file.
Ex.:
input File:
1
5
2
4
Output File:
1
25
4
16
Question (3) (Total: 100 points)
Write a program to read in two files. The first file contains the first name of a set of students. The
second file contains the last name of a set of students. Create a third file containing the full names of the
students.
Input Files: First Names and Last Names
First Names:
Jade
Chandler
Andy
Maggie
Last Names:
Moore
Anderson
Boateng
Bowles
Output File: Full Names
Full Names:
Jade Moore
Chandler Anderson
Andy Boateng
Maggie Bowles
Question (4) (Total: 100 points)
Write a program to read in the grades of three Cybersecurity courses (CYSE 250 CYSE 270 CYSE 200T)
of a set of students. Grades are separated by a space. Each line represents a student. Calculate the mean
grade of every student and write it into an output file.
Input File:
60 70 80
70 80 90
40 50 60
Output File:
70
80
50
Copy Files Python Program
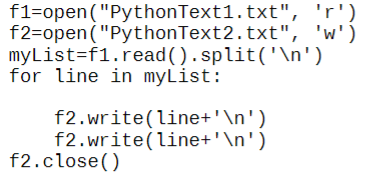
Square File Python Program
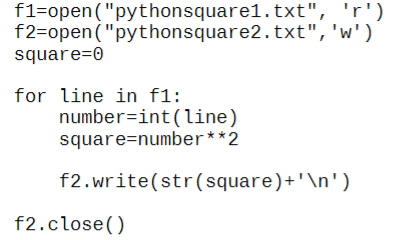
Full Names Python Program
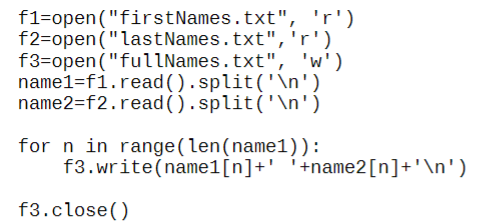
Test Averages Python Program
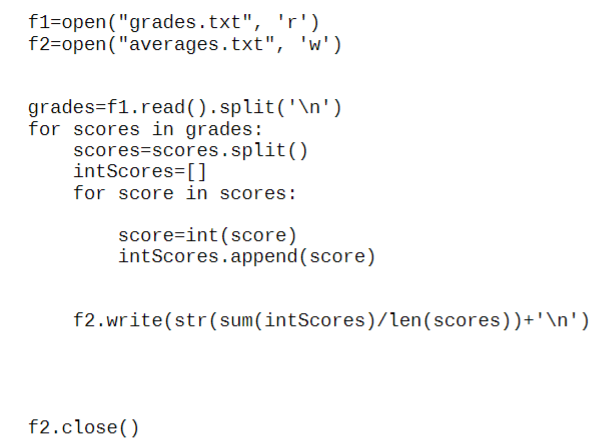
Final Project
Learning Objective: The group project will provide you with the opportunity to demonstrate
your understanding of the applied aspect of Python and socket programming in your
concentration.
1. The group project will be related to the contents of this course. A group of maximum two
students will develop and design their own project.
2. The group should find a related topic to socket programming.
3. To design the visual representation, you may choose general-purpose programming language
Python.
4. You need to present your work during the lecture time
5. You need to write and submit a project report (Maximum five-page, Font: Helvetica, Font
size: 12, single line inter-line spacing, page-setup: 1 inch all sides, excluding front page).
The report must include following sections:
• The problem statements.
• The details about the hardware (your machine) and software (include version) used in the
project.
• Software used to implement the project
• The results (Include screen shot of code and output) and discussions.
5. The internal documentation (i.e., comments) is required. You need to include Python code in
your project report as an appendix. However, the appendix will not be counted in the number of
pages of “Project Report”. It implies that appendix will have additional number of pages in the
project report. Additionally, there is no restriction of number of pages for the appendix.
6. Your code MUST include the following:
Loops
Functions
Lists and Dictionaries
Socket Programming
7. You must submit your report, presentation and code on Canvas.
Chore Incentive Project
For the full report with screenshots download the full file at the bottom of this text.
Chore Incentive Python Project
CYSE 250
Instructor
Hind AlDabagh
By Adam Haas
This program is an interactive game built to help incentivise my two children, 9 years old and 5 years old, to complete various chores each week without being asked. It will track their progress and offer a reward once enough chores have been completed. This python program project has been created and written with Intel(R) Core(TM) i7-4770 CPU @ 3.40GHz 3.40 GHz and is operating on the Windows 10 Pro operating system, using Python Version 3.11. This project is to demonstrate knowledge of Python code using loops, functions, lists, dictionaries, and socket programing. This program uses a server that runs in the background managing the score levels and prize winnings of the users. The client program will be the program that is used for interface and inputting chore completion and offering rewards when goals are reached. The server and client programs will regularly communicate for recording work completed, prize awards, and total scores.
This project is structured to help our family by encouraging self-motivation to earn future rewards. The way works is the children will enter their name that will coincide with a txt file that will record and track their scores. The program will update and record to the text file as chores are manually logged into the client program as seen in Figure 1.1 and then sent to the server program in Figure 1.2.
The chore options are set up together with the prize options in a list named Choices. The program differentiates between chores and prizes based on their value, choices are a positive number, while prizes are negative numbers. The choices can be observed in Figure 1.3.
The program will read from each childs text file to know whether they have enough points for a prize. If enough points are available for a prize they will be prompted to pick one, otherwise they will be prompted to keep doing chores. This can be observed on the client’s terminal in Figure 1.4.
This selection and check can be observed through this if, else statement and for loops in Figure 1.5. This will display on the client computer when users log on to see what options they have for entry.
If the user memorizes the name of the prizes a check is in place to prevent them from selecting a prize before they have enough points as seen in Figure 1.5.
Once the user responds to the server that a chore was completed. It will disconnect and be ready to be relaunched for more chore submissions. This program has a function created for it that automatically combines the earned points with previously awarded points called getTotalpoints as seen in Figure 1.6
Once the predetermined goal value is reached by a user. A message will print on the client computer. “Great job you have earned enough points for a prize. Please log back in to select a prize.The code for this can be observed in Figure 1.7 and the terminal display once the user logs back in can be viewed in Figure 1.8.
Each user will have a text file labeled with their name to track their progress and hold this information for future access and record. This can be seen in Figure 1.9 where the user Huck has completed a variety of chores and has selected a prize.
A separate text file is used to hold all prize data so the administrator isn’t challenged by reading through all completed work and prizes to find out what rewards need to be paid out. This can be observed in Figure 1.10.
This program uses a variety of Python coding techniques like loops, functions, lists, dictionaries, and socket programming to demonstrate my coding knowledge and understanding. The problem solved with this program creates a solution to a challenge that our family regularly faces by helping provide motivation for completion of chores by our children. It does this by requiring their self motivated work and receiving rewards..
Chore Incentive Server
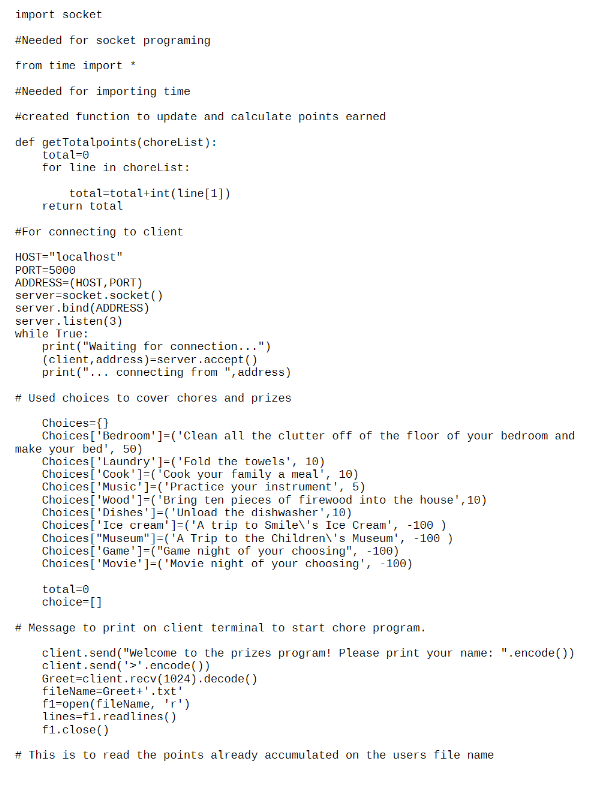
Chore Incentive Client
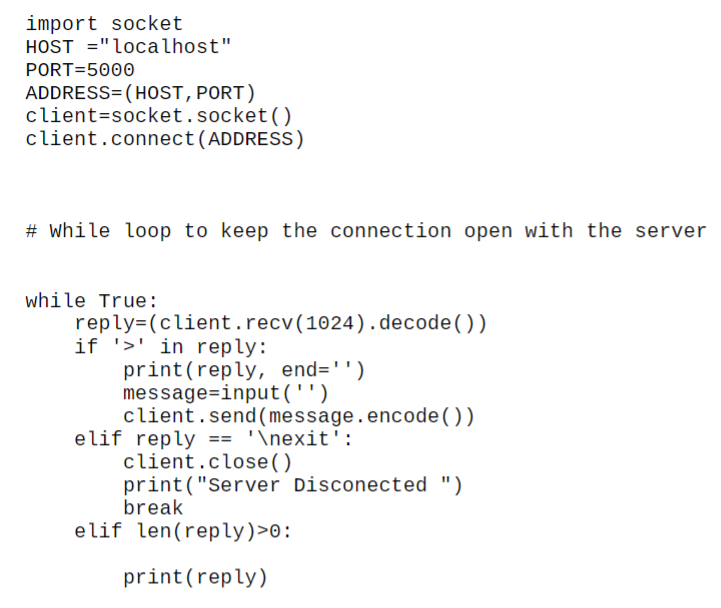