Question (1)
a. What is the significance of using function in python programming?
Functions are reusable and can perform specific tasks
b. What does the following statement do? You may execute this in Python IDE
and submit the screenshot for the output.
print(random.uniform(0.1, 0.5))
This function prints any random floating variable between the two given numbers (0.1
& 0.5)
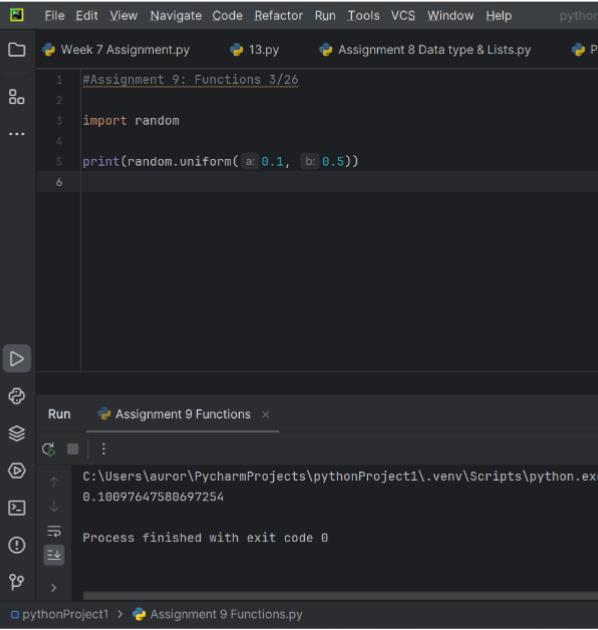
c. List five built-in functions in python.
Five built-in functions in python are max(), help(), sum(), input(), and print().
d. What is meant by scope of a variable in python (function)? Explain with an
example.
The scope refers to where the variable is available or able to be used.
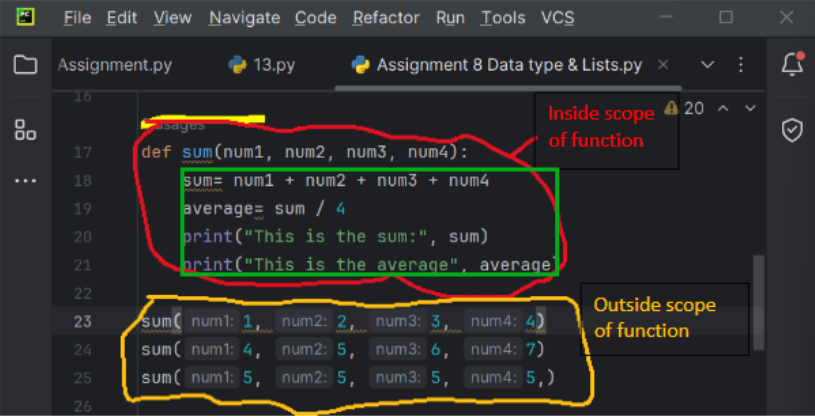
Question (2)
a. What is difference between list, Tuple and Dictionary in python?
All of these are collections of values. Lists and tuples are both ordered but unlike lists,
tuples cannot be changed. Dictionaries are unordered.
b. What is tuple in python? Explain with an example.
A tuple is much like a list however it uses () instead of [] and cannot be edited as shown in the
example.
Inside scope
of function
Outside scope
of function
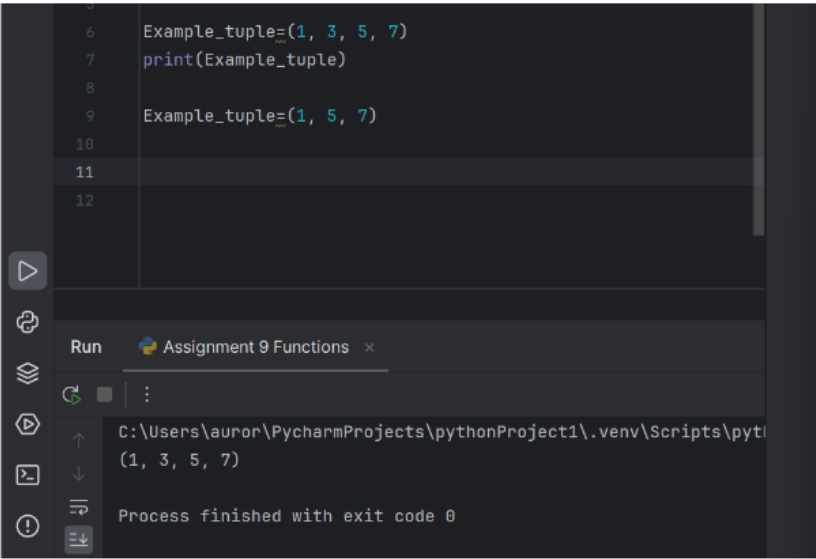
c. What is a module in python? How would you access a module in python?
Explain with an example.
A module is defined as a file with a python code like functions in it. All of the circles areas
from pycharm are different modules or files including python codes and functions.

Question (3)
a. Using/importing “math” library/module, use the built-in function (You
need to explore the function online) to find the largest item from a given list. Please
submit the screenshot for your code and its output.
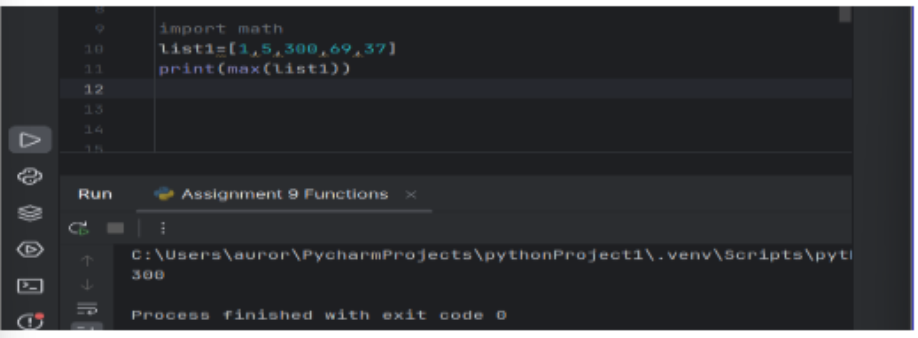
b. Write a function in python, that should do the following:
i) add(num1, num2): Returns the sum of two numbers num1 and num2.
ii) subtract(num1, num2): Returns the result of subtracting num2 from num1.
iii) multiply(num1, num2): Returns the product of two numbers num1 and num2.
Please submit the screenshot for your code and its output.
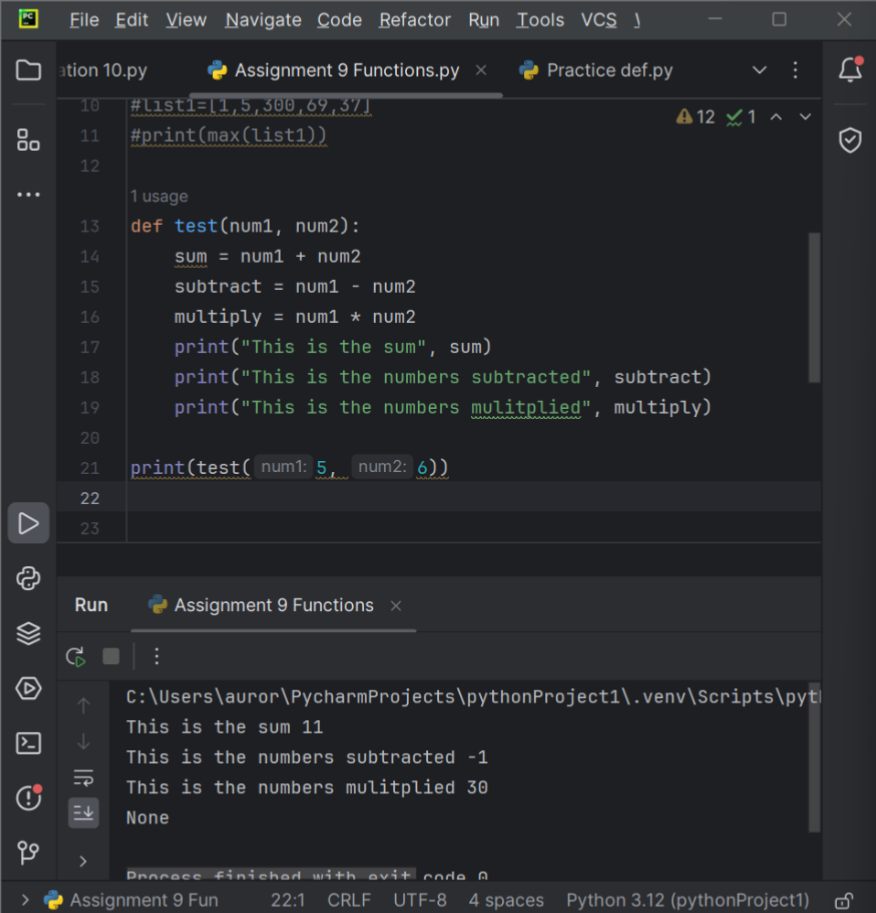